react-redux笔记
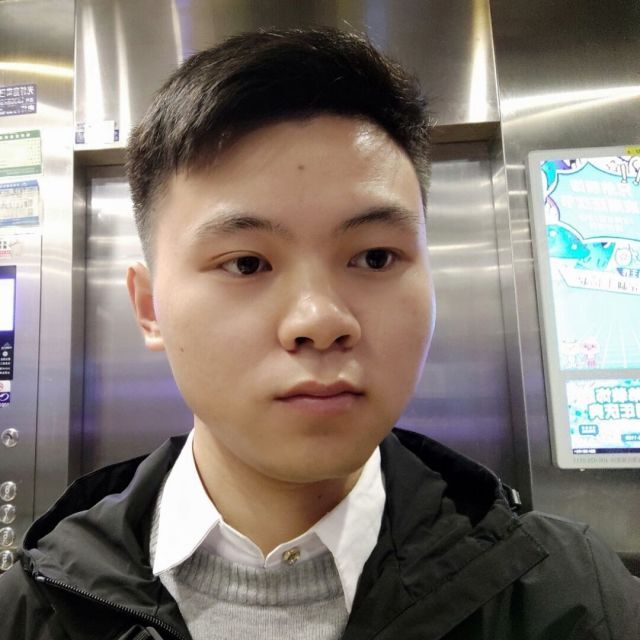
redux与react-redux使用
store.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19import { createStore } from 'redux';
const defaultState = {
list:['这是一个测试']
}
const reducer = (state = {...defaultState},action)=>{
const {type,payload} = action;
if(type ==='addItem'){
const obj = {...state};
obj.list = [...obj.list,payload]; //这里不能是obj.list.push(payload); 否则页面视图不更新
return obj;
}
return state;
}
const store = createStore(reducer); //设置默认值,也可以通过createStore来指定第二个参数
/*
如
const store = createStore(reducer,defaultState,appMiddleware(thunk))
*/
export default store;根组件 index.jsx
1
2
3
4
5
6
7
8
9import { Provider } from 'react-redux';
import Store from './store';
root.render(
<React.StrictMode>
<Provider store={Store}>
<App />
</Provider>
</React.StrictMode>
);在需要使用的地方使用connect封装为高阶组件
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51class InputGroup extends React.Component{
constructor(props){
super(props);
}
state = {
name:'yuhong',
age:22,
count:11
}
submit = ()=>{
const value = this.input.value;
this.props.handleList(value)
}
render(){
return (<div>
<input type="text" ref={el=>this.input = el}/><button onClick={this.submit}>提交</button>
{JSON.stringify(this.props.list)}
</div>)
}
}
const mapStateToProps = state=>{
return {
list:state.list
}
}
/**
mapDispatchToProps有两种写法,一种是申明是直接对象 -------[首选]
**/
const mapDispatchToProps = {
handleList(payload){
return {
type:'addItem',
payload
}
}
}
/**
另外一种是写state相同,是一个函数,此时拥有dispatch参数
**/
const mapDispatchToProps = dispatch=>{
return {
handleList(payload){
dispatch({
type:'addItem',
payload
})
}
}
}
export default connect(mapStateToProps,mapDispatchToProps)(InputGroup);
总结为createStore时,从redux中引入,而Provider 或者 connect是从react-redux中引入
redux-thunk中间件
使用
1 | import { createStore,applyMiddleware } from 'redux' |
在需要使用的地方mapDispatchToProps中返回一个对象,变为返回一个函数,函数接收一个参数dispatch
1 | const mapDispatchToProps = { |
数据持久化
官方文档:https://www.npmjs.com/package/redux-persist
将会把store中的state通过localstroge缓存 在浏览器中,如果需要清除时,可以使用localstorge.removeItem()去重置
1 | import { createStore } from 'redux' |
redux装饰器
安装
1 | yarn add @babel/plugin-proposal-decorators |
修改package.json
1 | "babel": { |
使用
1 | @connect( |
react支持@connect装饰器写法,详细搜索
- 本文标题:react-redux笔记
- 本文作者:邵预鸿
- 创建时间:2022-06-02 17:32:25
- 本文链接:/images/logo.jpg2022/06/02/react-redux笔记/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!