vue将组件封装为方法调用
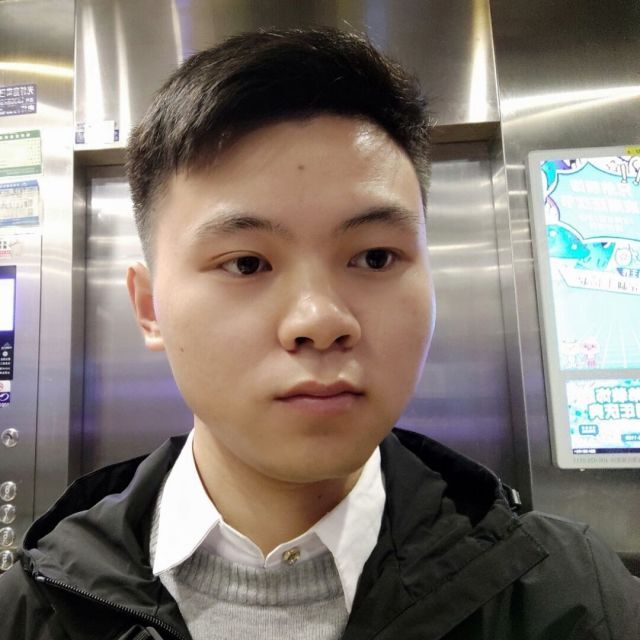
v-bind绑定对象
1 | <!-- 绑定一个全是 attribute 的对象 --> |
某个组件
1 | <template> |
注册为全局方法
1 | import Dialog from './yuhong.vue'; |
main.js 使用vue.use
1 | Vue.use(install,{传递的数据}) |
使用
this.$yu.config({ option:{},text: ‘1’ }).visible(true)
方法2,推荐
首先封装一个组件
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35<template>
<section class="dialog">
<img src="https://img01.yzcdn.cn/vant/apple-3.jpg" style="width: 200px"/>
<p>{{msg}}</p>
<div>
<button @click="onCancelFunc">返回</button>
<button @click="onSaveFunc">确定</button>
</div>
</section>
</template>
<script>
export default {
props: ['onClose','onSave'],
data(){
return {
show: true
}
},
mounted(){
console.log(this);
},
methods:{
onCancelFunc(){
this.$emit('onTest','我爱李泰利'); // 通过emit提交事件
this.onClose && this.onClose(); // 通过父组件传入的回调函数提交 事件
},
onSaveFunc(){
this.onSave && this.onSave()
}
}
}
</script>传组件使用类,封装
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36import VantModal from './vant-modal.vue';
import Vue from 'vue';
class Modal{
constructor(props){
this.app = null;
this.mountElement = null;
this.show(props);
}
show(props = {}){
const oDiv = document.createElement('div');
oDiv.className = props.custormClassName || 'yh-dialog';
const oChildDiv = document.createElement('div');
oDiv.appendChild(oChildDiv);
document.body.appendChild(oDiv);
this.app = new Vue({
render: h => h(VantModal, {
props,
attrs: props,
}),
}).$mount(oChildDiv);
this.mountElement = oDiv;
// 接收子组件的emit事件
this.app.$children[0].$on('onTest', data=>{
console.log('modal.js中收到 自定义事件onTest',data);
props.onTest(data);
})
}
close(props = {}){
this.app.$destroy();
document.body.removeChild(this.mountElement);
}
}
export default Modal;调用
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37<template>
<div>
<button @click="openMask">打开警告弹窗</button>
</div>
</template>
<script>
import Modal from './modal'
export default {
data(){
return {
}
},
methods:{
openMask(){
const app = new Modal({
// msg: '我通过命令式渲染',
onClose:()=>{
console.log('关闭')
app.close();
},
onSave: ()=>{
console.log('保存')
app.close();
},
onTest: (data)=>{
console.log('ontest is start', data);
}
});
},
}
}
</script>
- 本文标题:vue将组件封装为方法调用
- 本文作者:邵预鸿
- 创建时间:2023-03-02 10:06:49
- 本文链接:/images/logo.jpg2023/03/02/vue将组件封装为方法调用/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!